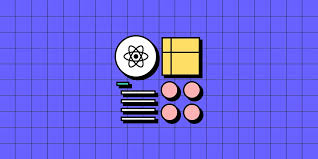
Ace System Design Interview
- Published on
- Authors
- Author
- Ram Simran G
- twitter @rgarimella0124
System design interviews can be daunting, especially for those new to the field or transitioning into more senior roles. However, with a structured approach, you can navigate these interviews with confidence. This guide breaks down the process into seven manageable steps, helping you tackle even the most complex system design problems.
Step 1: Clarify Requirements
The foundation of any good system design is a clear understanding of what you’re building. Don’t rush into solutions; take the time to gather all necessary information.
Functional Requirements:
- What are the core features the system should support?
- Who are the users, and how will they interact with the system (web, mobile app, API, etc.)?
- What key data types must the system handle (text, images, structured data, etc.)?
Non-functional Requirements:
- What’s the expected size of the user base?
- What’s the anticipated read/write ratio?
- Is consistency or availability more critical for this system?
- What are the latency and throughput requirements?
Example: For a social media platform, you might clarify that users need to post updates, follow others, and receive a personalized feed. Non-functionally, you might determine that the system needs to support millions of users with a read-heavy workload and low latency for feed generation.
Step 2: Capacity Estimation
Once you understand the requirements, estimate the system’s scale. This step helps inform your design decisions and highlights potential bottlenecks.
- Calculate expected reads/writes per second
- Estimate required database and cache storage
- Determine necessary network bandwidth
Example: For our social media platform, we might estimate 100,000 daily active users, each making 20 reads and 2 writes per day, leading to about 23 reads/second and 2.3 writes/second at peak times.
Step 3: High-Level Design
Sketch out the system’s architecture, identifying major components and their interactions.
- Draw a block diagram of the system
- Identify key components: databases, servers, clients, load balancers, CDN, cache, message queues, etc.
Example: Our social media platform might include web/mobile clients, API servers, a relational database for user data, a NoSQL database for posts, a caching layer, and a CDN for static content.
Step 4: Database Design
Choose the appropriate database type and design the schema based on your data requirements.
- Decide between SQL, NoSQL, or a combination
- Define the database schema, including tables/collections and their relationships
Example: We might use PostgreSQL for user data and relationships, and Cassandra for storing and retrieving posts efficiently.
Step 5: API Design
Design the interfaces through which clients will interact with your system.
- Choose an appropriate API style (REST, GraphQL, gRPC, etc.)
- List and define key API endpoints
- Specify request/response formats
Example:
POST /api/v1/posts
{
"user_id": "123",
"content": "Hello, world!",
"media_url": "https://example.com/image.jpg"
}
Step 6: Dive into Key Components
Discuss each major component in detail, explaining its role, implementation, and interactions.
- Elaborate on core features and services
- Explain how components scale and perform under load
- Discuss data flow between components
Example: For the feed generation service, explain how it retrieves followed users’ posts, applies ranking algorithms, and caches results for quick retrieval.
Step 7: Address Key Issues: Scalability, Reliability, and Security
Finally, demonstrate your system’s robustness by addressing critical concerns:
- Scalability: Discuss sharding, replication, and partitioning strategies
- Caching: Identify where and how to implement caching effectively
- Fault Tolerance: Explain strategies for handling component failures
- Security: Address authentication, authorization, and data protection
Example: Implement database sharding based on user_id to distribute load. Use read replicas to scale read operations. Implement a distributed caching layer using Redis to reduce database load for frequently accessed data.
Cheers,
Sim