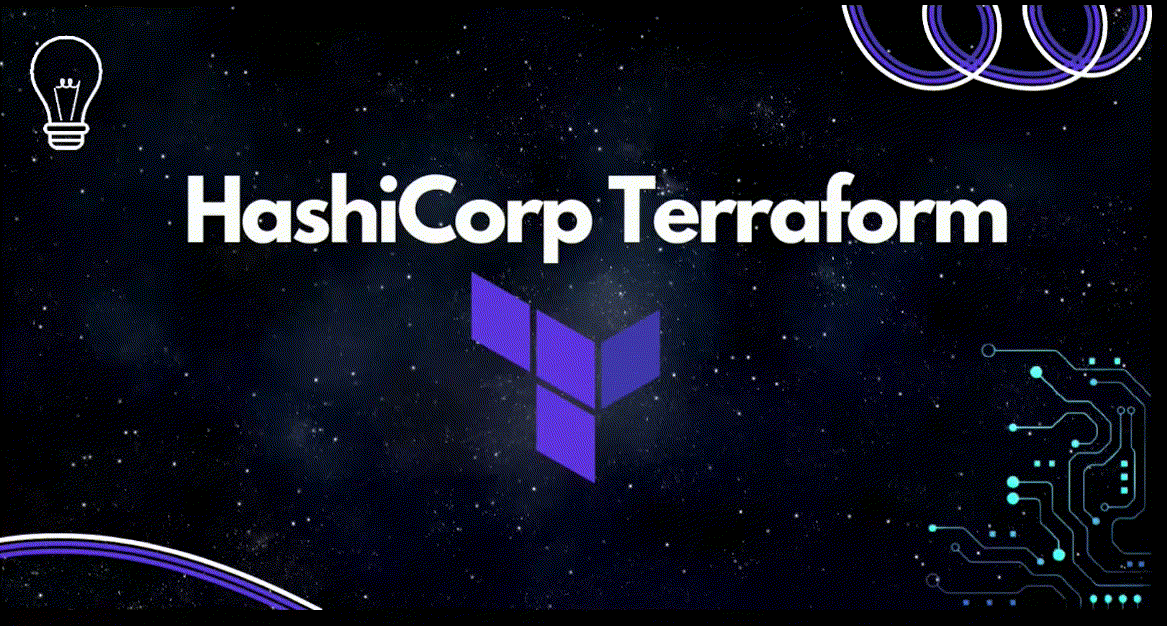
Monitoring with Terraform
- Published on
- Authors
- Author
- Ram Simran G
- twitter @rgarimella0124
In the world of Infrastructure as Code (IaC), Terraform has emerged as a powerful tool for provisioning and managing cloud resources. However, deploying infrastructure is only half the battle; monitoring that infrastructure is crucial for ensuring its health, performance, and security. In this comprehensive guide, we’ll explore how Terraform can be leveraged to set up robust monitoring solutions, integrating seamlessly with your infrastructure management workflow.
Introduction to Monitoring in IaC
Infrastructure as Code has revolutionized the way we deploy and manage cloud resources. With tools like Terraform, we can version, test, and automate our infrastructure deployments. However, the dynamic nature of cloud environments necessitates robust monitoring solutions to ensure that our infrastructure operates as expected.
Monitoring in the context of IaC involves:
- Resource health checks
- Performance metrics collection
- Log aggregation and analysis
- Alerting and notification systems
- Security and compliance auditing
By incorporating monitoring into our IaC workflows, we can achieve:
- Proactive issue detection: Identify and address problems before they impact users.
- Performance optimization: Gain insights to fine-tune resource allocation and application performance.
- Cost management: Track resource usage to optimize spending.
- Compliance and security: Ensure infrastructure adheres to security policies and compliance requirements.
- Continuous improvement: Use monitoring data to inform infrastructure evolution and optimization.
Terraform’s Role in Monitoring
Terraform’s strength lies in its ability to define and manage infrastructure resources declaratively. When it comes to monitoring, Terraform can:
- Provision monitoring resources: Create and manage monitoring-specific infrastructure like log storage buckets, metrics databases, and dashboards.
- Configure monitoring agents: Deploy and configure monitoring agents on compute resources.
- Set up alerting rules: Define alerting thresholds and notification channels.
- Manage access controls: Configure IAM roles and permissions for monitoring services.
- Integrate with existing tools: Set up integrations with popular monitoring platforms.
By using Terraform to manage both your core infrastructure and monitoring setup, you ensure consistency and reduce the risk of configuration drift between environments.
Setting Up Basic Monitoring with Terraform
Let’s start with a basic example of setting up monitoring for an AWS EC2 instance using Terraform and AWS CloudWatch.
# Define the AWS provider
provider "aws" {
region = "us-west-2"
}
# Create an EC2 instance
resource "aws_instance" "web_server" {
ami = "ami-0c55b159cbfafe1f0"
instance_type = "t2.micro"
tags = {
Name = "WebServer"
}
}
# Create a CloudWatch metric alarm
resource "aws_cloudwatch_metric_alarm" "high_cpu_utilization" {
alarm_name = "high-cpu-utilization"
comparison_operator = "GreaterThanThreshold"
evaluation_periods = "2"
metric_name = "CPUUtilization"
namespace = "AWS/EC2"
period = "120"
statistic = "Average"
threshold = "80"
alarm_description = "This metric monitors ec2 cpu utilization"
alarm_actions = [aws_sns_topic.alerts.arn]
dimensions = {
InstanceId = aws_instance.web_server.id
}
}
# Create an SNS topic for alerts
resource "aws_sns_topic" "alerts" {
name = "high-cpu-alert"
}
# Create an SNS topic subscription
resource "aws_sns_topic_subscription" "email_alerts" {
topic_arn = aws_sns_topic.alerts.arn
protocol = "email"
endpoint = "alerts@example.com"
}
This example demonstrates:
- Creating an EC2 instance
- Setting up a CloudWatch alarm to monitor CPU utilization
- Creating an SNS topic for alerts
- Configuring an email subscription for the SNS topic
With this configuration, you’ll receive an email alert when the CPU utilization of your EC2 instance exceeds 80% for two consecutive 2-minute periods.
Advanced Monitoring Techniques with Terraform
As your infrastructure grows more complex, so too will your monitoring needs. Here are some advanced techniques for monitoring with Terraform:
1. Custom Metrics and Logs
You can use Terraform to set up custom metrics and log collection:
# Create a CloudWatch log group
resource "aws_cloudwatch_log_group" "app_logs" {
name = "/app/production"
retention_in_days = 30
}
# Create a custom metric filter
resource "aws_cloudwatch_log_metric_filter" "error_count" {
name = "ErrorCount"
pattern = "ERROR"
log_group_name = aws_cloudwatch_log_group.app_logs.name
metric_transformation {
name = "ErrorCount"
namespace = "CustomMetrics"
value = "1"
}
}
# Create an alarm based on the custom metric
resource "aws_cloudwatch_metric_alarm" "high_error_rate" {
alarm_name = "high-error-rate"
comparison_operator = "GreaterThanThreshold"
evaluation_periods = "1"
metric_name = "ErrorCount"
namespace = "CustomMetrics"
period = "300"
statistic = "Sum"
threshold = "10"
alarm_description = "This metric monitors error count in logs"
alarm_actions = [aws_sns_topic.alerts.arn]
}
This configuration creates a log group, sets up a metric filter to count ERROR occurrences in logs, and creates an alarm based on this custom metric.
2. Distributed Tracing
For microservices architectures, distributed tracing is crucial. You can use Terraform to set up tracing infrastructure:
# Set up AWS X-Ray
resource "aws_xray_sampling_rule" "xray_sampling" {
rule_name = "Default"
priority = 1
reservoir_size = 1
fixed_rate = 0.05
url_path = "*"
host = "*"
http_method = "*"
service_type = "*"
service_name = "*"
resource_arn = "*"
}
# Enable X-Ray tracing for API Gateway
resource "aws_api_gateway_stage" "example" {
deployment_id = aws_api_gateway_deployment.example.id
rest_api_id = aws_api_gateway_rest_api.example.id
stage_name = "prod"
xray_tracing_enabled = true
}
This setup enables X-Ray tracing for your API Gateway, allowing you to trace requests as they flow through your microservices.
3. Infrastructure-wide Monitoring
For a holistic view of your infrastructure, you can use Terraform to set up dashboards:
resource "aws_cloudwatch_dashboard" "main" {
dashboard_name = "main-dashboard"
dashboard_body = jsonencode({
widgets = [
{
type = "metric"
x = 0
y = 0
width = 12
height = 6
properties = {
metrics = [
["AWS/EC2", "CPUUtilization", "InstanceId", aws_instance.web_server.id]
]
period = 300
stat = "Average"
region = "us-west-2"
title = "EC2 Instance CPU"
}
},
{
type = "log"
x = 0
y = 6
width = 24
height = 6
properties = {
query = "fields @timestamp, @message | filter @message like /ERROR/"
region = "us-west-2"
title = "Error Logs"
view = "table"
}
}
]
})
}
This creates a CloudWatch dashboard with two widgets: one showing EC2 CPU utilization and another displaying error logs.
Integrating Popular Monitoring Tools
While cloud-native monitoring solutions are powerful, many organizations use third-party monitoring tools. Terraform can help integrate these tools into your infrastructure:
Prometheus and Grafana
resource "helm_release" "prometheus" {
name = "prometheus"
repository = "https://prometheus-community.github.io/helm-charts"
chart = "prometheus"
namespace = "monitoring"
set {
name = "server.persistentVolume.enabled"
value = "false"
}
}
resource "helm_release" "grafana" {
name = "grafana"
repository = "https://grafana.github.io/helm-charts"
chart = "grafana"
namespace = "monitoring"
set {
name = "persistence.enabled"
value = "true"
}
set {
name = "persistence.size"
value = "10Gi"
}
}
This Terraform configuration uses the Helm provider to deploy Prometheus and Grafana to a Kubernetes cluster.
Datadog
provider "datadog" {
api_key = var.datadog_api_key
app_key = var.datadog_app_key
}
resource "datadog_monitor" "cpu_monitor" {
name = "CPU usage alert"
type = "metric alert"
message = "CPU usage is above 80%"
query = "avg(last_5m):avg:system.cpu.user{*} by {host} > 80"
notify_no_data = false
require_full_window = true
monitor_thresholds {
critical = 80
warning = 70
}
notify_audit = false
timeout_h = 0
include_tags = true
tags = ["env:production", "app:web"]
}
This example sets up a Datadog monitor for CPU usage using the Datadog Terraform provider.
Best Practices for Monitoring with Terraform
Use modules: Create reusable Terraform modules for common monitoring patterns to ensure consistency across your infrastructure.
Leverage tags: Use resource tagging to organize and categorize your monitoring resources, making them easier to manage and update.
Separate concerns: Keep your monitoring configuration separate from your main infrastructure code to allow for independent updates and management.
Version control: Store your Terraform monitoring configurations in version control to track changes and facilitate collaboration.
Use variables: Parameterize your monitoring configurations to make them flexible and reusable across different environments.
Implement least privilege: Use IAM roles and policies to ensure your monitoring setup has only the permissions it needs.
Regular updates: Keep your Terraform providers and modules up to date to leverage new features and security improvements.
Testing: Implement automated testing for your Terraform configurations to catch issues before they reach production.
Challenges and Solutions
While using Terraform for monitoring brings many benefits, there are also challenges to consider:
State management: As your monitoring infrastructure grows, managing Terraform state becomes more complex. Consider using remote state storage and state locking to facilitate team collaboration.
Performance: Large Terraform configurations can be slow to apply. Use
-target
flags or split your configuration into smaller, more manageable pieces.Secret management: Avoid hardcoding sensitive data like API keys in your Terraform files. Use tools like HashiCorp Vault or AWS Secrets Manager to securely manage secrets.
Cross-cloud monitoring: If you’re using multiple cloud providers, consider using a cloud-agnostic monitoring solution or creating abstraction layers in your Terraform code.
Drift detection: Regularly run
terraform plan
to detect and address any drift between your defined configuration and the actual state of your infrastructure.
Future of Monitoring with Terraform
As Terraform and the broader IaC ecosystem evolve, we can expect to see:
AI-driven monitoring: Integration of machine learning algorithms to predict and prevent issues before they occur.
Improved visualization: Enhanced capabilities for creating and managing complex dashboards and visualizations directly through Terraform.
Serverless monitoring: Better support for monitoring serverless and ephemeral infrastructure.
Cross-cloud standardization: More standardized approaches to monitoring across different cloud providers.
IoT and edge computing: Expanded capabilities for monitoring distributed systems, including IoT devices and edge computing nodes.
Conclusion
Monitoring is a critical aspect of managing modern infrastructure, and Terraform provides powerful tools to integrate monitoring into your Infrastructure as Code workflows. By leveraging Terraform for both infrastructure provisioning and monitoring setup, you can ensure consistency, improve collaboration, and create more resilient systems.
As you embark on your journey of monitoring with Terraform, remember that it’s an iterative process. Start with basic monitoring, gradually incorporate more advanced techniques, and continuously refine your approach based on the specific needs of your infrastructure and applications.
By following the best practices and techniques outlined in this guide, you’ll be well-equipped to create a robust, scalable, and efficient monitoring solution that grows with your infrastructure. Happy monitoring!
Cheers,
Sim